Introduction
When I deployed a Blog module in a Magento 2 Shop, I was wondering why It didn’t populated all the shown fields in the corresponding backend Admin View. Namely the Created At
, Modified At
and the Custom Date
field were showing blank values, despite having valid values in the database.
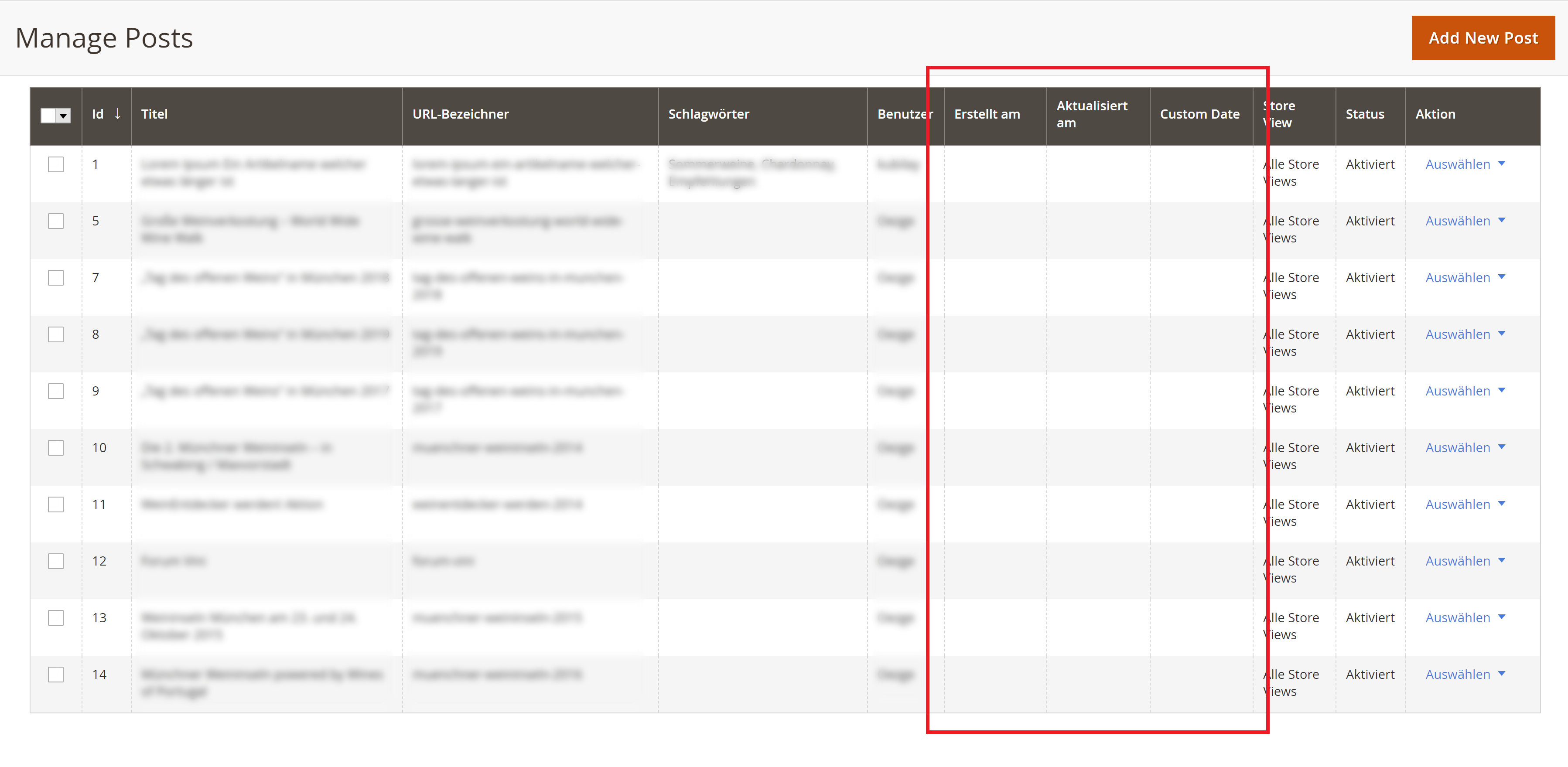
Short side note: The Custom Date
Field was added manually as the name may be imply – See here for a how-to. But now back to the topic:
This article will present a summary of a successful method to bring back the correct values to the empty UI Component Fields.
Looking for Bugs and Mistakes
The first place where to look for the issue is the place where the actual fields are defined. Namely the responsible XML File. In my case that is: /app/code/EeroKaan/Blog/view/adminhtml/ui_component/post_listing.xml
<dataSource name="post_listing_data_source">
<argument name="dataProvider" xsi:type="configurableObject">
<argument name="class" xsi:type="string">PostGridDataProvider</argument>
<argument name="name" xsi:type="string">post_listing_data_source</argument>
<argument name="primaryFieldName" xsi:type="string">post_id</argument>
<argument name="requestFieldName" xsi:type="string">post_id</argument>
<argument name="data" xsi:type="array">
<item name="config" xsi:type="array">
<item name="update_url" xsi:type="url" path="mui/index/render"/>
</item>
</argument>
</argument>
<argument name="data" xsi:type="array">
<item name="js_config" xsi:type="array">
<item name="component" xsi:type="string">Magento_Ui/js/grid/provider</item>
</item>
</argument>
</dataSource>
<column name="created_at" class="Magento\Ui\Component\Listing\Columns\Date">
<argument name="data" xsi:type="array">
<item name="config" xsi:type="array">
<item name="component" xsi:type="string">Magento_Ui/js/grid/columns/date</item>
<item name="filter" xsi:type="string">dateRange</item>
<item name="visible" xsi:type="boolean">true</item>
<item name="dataType" xsi:type="string">date</item>
<item name="dateFormat" xsi:type="string">yyyy-MM-dd HH:mm:ss</item>
<item name="label" xsi:type="string" translate="true">Created At</item>
<item name="sortOrder" xsi:type="number">120</item>
</item>
</argument>
</column>
<column name="updated_at" class="Magento\Ui\Component\Listing\Columns\Date">
<argument name="data" xsi:type="array">
<item name="config" xsi:type="array">
<item name="component" xsi:type="string">Magento_Ui/js/grid/columns/date</item>
<item name="filter" xsi:type="string">dateRange</item>
<item name="visible" xsi:type="boolean">true</item>
<item name="dataType" xsi:type="string">date</item>
<item name="dateFormat" xsi:type="string">yyyy-MM-dd HH:mm:ss</item>
<item name="label" xsi:type="string" translate="true">Updated At</item>
<item name="sortOrder" xsi:type="number">130</item>
</item>
</argument>
</column>
<column name="eero_custom_date" class="Magento\Ui\Component\Listing\Columns\Date">
<argument name="data" xsi:type="array">
<item name="config" xsi:type="array">
<item name="component" xsi:type="string">Magento_Ui/js/grid/columns/date</item>
<item name="filter" xsi:type="string">dateRange</item>
<item name="visible" xsi:type="boolean">true</item>
<item name="dataType" xsi:type="string">date</item>
<item name="dateFormat" xsi:type="string">yyyy-MM-dd HH:mm:ss</item>
<item name="label" xsi:type="string" translate="true">Custom Date</item>
<item name="sortOrder" xsi:type="number">140</item>
</item>
</argument>
</column>
In Line 20 you can see the DataProvider class PostGridDataProvider
being defined. Following the rabbit-hole the /app/code/EeroKaan/Blog/etc/di.xml
File tells us, what the PostGridDataProvider
class actually is:
<virtualType name="PostGridDataProvider" type="EeroKaan\Blog\Ui\DataProvider\Post\PostDataProvider">
<arguments>
<argument name="collection" xsi:type="object" shared="false">EeroKaan\Blog\Model\Resource\Post\Collection</argument>
<argument name="filterPool" xsi:type="object" shared="false">PostGirdFilterPool</argument>
</arguments>
</virtualType>
Line 60 links the PostGridDataProvider
to the EeroKaan\Blog\Ui\DataProvider\Post\PostDataProvider
class. Inside of the PostDataProvider
class under the /app/code/EeroKaan/Blog/Ui/DataProvider/Post/PostDataProvider.php
path, we can see a certain getData()
Method. By inserting a print_r( $items )
after the initialisation of the collection we can output the collection array itself.
public function getData() {
if (!$this->getCollection()->isLoaded()) {
$this->getCollection()->load();
}
$items = $this->getCollection()->toArray();
print_r( $items );
return [
'totalRecords' => $this->getCollection()->getSize(),
'items' => array_values($items['items']),
];
}
[items] => Array
(
[0] => Array
(
[post_id] => 1
[title] => Lorem Ipsum A B C
[url_key] => lorem-ipsum-a-b-c
[thumbnail] => blog/t/e/test-576x432_1.jpg
[image] => blog/t/e/test-576x432_3.jpg
[short_content] => <p>This is the Short Content Text</p>
[content] => <p><img src="{{media url="blog/eero-test-image-01.jpg"}}" width="1920" height="1080"></p>
[meta_keywords] =>
[meta_description] =>
[tags] => Test, Article, EeroLorem, Ipsum
[user] => Eero
[updated_by_user] => Eero
[status] => 1
[_first_store_id] => 1
[store_code] => eero_kaan_shop
[store_id] => Array
(
[0] => 0
)
As you can see from the data the array holds, the Admin view doesn’t have any chance to display the requested data, since it’s not present. The fields created_at
, updated_at
and eero_custom_date
are missing. To add them to the array anyways we can now proceed with the actual solution.
Fix the Code in the Right Place
To add the missing fields to the collection, we utilize the simple addField()
Method in the Class Constructor like so:
public function __construct(
$name,
$primaryFieldName,
$requestFieldName,
CollectionFactory $collectionFactory,
array $addFieldStrategies = [],
array $addFilterStrategies = [],
array $meta = [],
array $data = []
)
{
parent::__construct($name, $primaryFieldName, $requestFieldName, $meta, $data);
$this->collection = $collectionFactory->create();
$this->addFieldStrategies = $addFieldStrategies;
$this->addFilterStrategies = $addFilterStrategies;
$this->addField("created_at");
$this->addField("updated_at");
$this->addField("eero_custom_date");
}
Then checking again with the print_r()
Method:
[items] => Array
(
[0] => Array
(
[created_at] => 2019-08-17 20:20:08
[updated_at] => 2020-03-03 12:02:02
[eero_custom_date] => 2020-02-10 01:00:00
[post_id] => 1
[title] => Lorem Ipsum A B C
[url_key] => lorem-ipsum-a-b-c
[thumbnail] => blog/t/e/test-576x432_1.jpg
[image] => blog/t/e/test-576x432_3.jpg
[short_content] => <p>This is the Short Content Text</p>
[content] => <p><img src="{{media url="blog/eero-test-image-01.jpg"}}" width="1920" height="1080"></p>
[meta_keywords] =>
[meta_description] =>
[tags] => Test, Article, EeroLorem, Ipsum
[user] => Eero
[updated_by_user] => Eero
[status] => 1
[_first_store_id] => 1
[store_code] => eero_kaan_shop
[store_id] => Array
(
[0] => 0
)
Since the necessary fields are now present, the Admin UI Component is now able to display our field values:
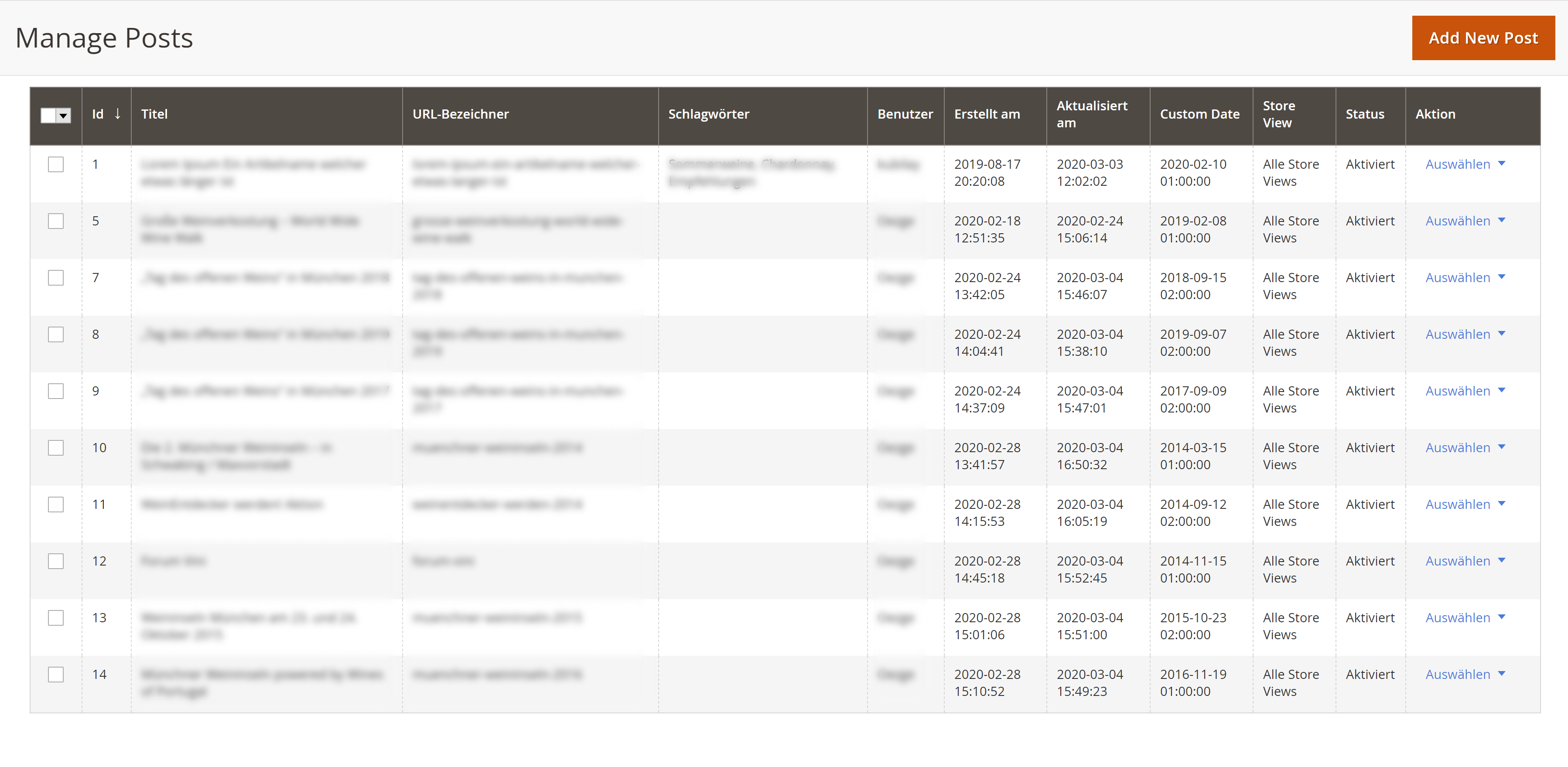
And there you have it: Thats how I was able to finally implement the missing values in my Magento 2 Admin View. Hopefully this way is also applicable to your case.